Using the Chain of Responsibility Design Pattern in Java
Table Of Content
The chain will process the request in the same order as below image. Note that we can implement this solution easily in a single program itself but then the complexity will increase and the solution will be tightly coupled. So we will create a chain of dispense systems to dispense bills of 50$, 20$ and 10$. Create a class file named ProjectLeader.cs and copy and paste the following code. As you can see, the MAX_LEAVES_CAN_APPROVE variable holds the maximum leave value the Project Leader can approve. As part of the ApplyLeave method, we check whether the Project Leader can approve the number of leaves the employee applies.
Using the Chain of Responsibility Design Pattern in Java
While it has advantages like flexibility and decoupling, be mindful of potential downsides, such as the risk of unhandled requests and slight performance overhead. Ultimately, the Chain of Responsibility pattern empowers you to design elegant and modular solutions to complex problems in the world of software engineering. Design patterns are indispensable tools in the software engineer’s toolbox. The Chain of Responsibility pattern is a powerful choice when you need to create a flexible and extensible processing chain. By understanding its principles and applying them in Python, you can improve the maintainability and scalability of your software systems. Imagine having a toolbox of tried-and-true solutions readily available.
Participants in the solution
In this series, we’ll explore what these patterns are and how they can elevate your coding skills. In this pattern, normally each receiver contains reference to another receiver. If one object cannot handle the request then it passes the same to the next receiver and so on.
UML class and sequence diagram
It fosters a more flexible and modular system by enabling tasks to be efficiently divided and executed without the need for requesters to know the precise details of their handling. Create a class file named HR.cs and copy and paste the following code. As you can see, the MAX_LEAVES_CAN_APPROVE variable holds the maximum leave value the HR can approve. As part of the ApplyLeave method, we check whether HR approves the leave. If not, then it will print that your leave application is suspended. If the CoR is stressed by several requests and the handlers are also complex – maybe they create other classes to process the request – a Service Container could be useful.
Design Patterns in Python: Adapter
While the example below doesn’t solve all the CoR issues, it shows how the pattern can be easily restructured and adapted to different logic. If you are interested, you can use the following GitHub link to see my complete implementation of Chain of Responsibility pattern. As you can see on the UML diagram, it has 3 type of components named as, Client, Handler, and Concrete-Handler. When a user points the mouse cursor at an element and presses the F1 key, the application detects the component under the pointer and sends it a help request.
Best of 2023: Top 9 Microservices Design Patterns - Cloud Native Now
Best of 2023: Top 9 Microservices Design Patterns.
Posted: Wed, 03 Jan 2024 08:00:00 GMT [source]
Decoupling The Object Creation
When we insert our ATM card into the machine and initiate a transaction, the ATM system employs the Chain of Responsibility pattern to handle our request, ultimately providing us with the requested cash. Now we have to implement a separate class for each request approver (Concrete Handlers) in this company. As a result, I have created a separate class for Team Leader, Project Leader, HR, and Manager. All these classes extend the “LeaveHandler” class and implement the “applyLeave” method within them. The Cocoa and Cocoa Touch frameworks, used for OS X and iOS applications respectively, actively use the chain-of-responsibility pattern for handling events. Objects that participate in the chain are called responder objects, inheriting from the NSResponder (OS X)/UIResponder (iOS) class.
Diagrammatical Representation of the Example
The application can attempt to authenticate a user to the system whenever it receives a request that contains the user’s credentials. However, if those credentials aren’t correct and authentication fails, there’s no reason to proceed with any other checks. The request is sent by the client, who then forwards it to the chain’s first handler.
The request bubbles up through all the element’s containers until it reaches the element that’s capable of displaying the help information. Eventually, the operator passes your call to one of the engineers, who had probably longed for a live human chat for hours as he sat in his lonely server room in the dark basement of some office building. The engineer tells you where to download proper drivers for your new hardware and how to install them on Linux.
Within the “Leave” class I have defined fields like “numberOfDays”, “empTier”, and “reason.” Those are the facts, that the company going to consider before approving the leave request. Since you can link the handlers in the chain in any order, all requests will get through the chain exactly as you planned. For example, the Dialog class, which renders the main window of the app, would be the root of the object tree.
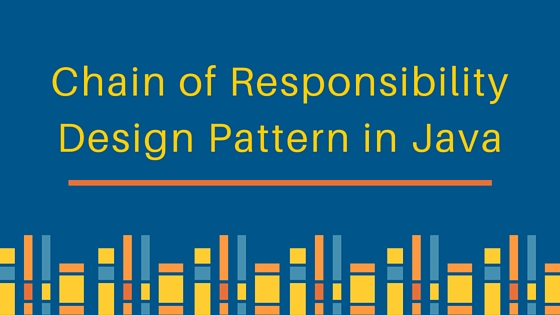
To avoid this, it’s important to have a termination condition or a default handler at the end of the chain. In conclusion, the Chain of Responsibility pattern is a powerful and elegant design pattern used in various software scenarios. Its ability to manage and conditionally propagate requests in a filter chain makes it a valuable tool in the software architect’s toolbox. If an employee requests a leave for 14 days or less, the Team leader cannot handle that request, since he only has authority to approve a leave up to 7 days. In that case the request will be pass to the Project Leader, who have authority to handle that request.
The association of corporate social responsibility and sustainable consumption and production patterns: The mediating ... - ScienceDirect.com
The association of corporate social responsibility and sustainable consumption and production patterns: The mediating ....
Posted: Tue, 15 Aug 2023 07:00:00 GMT [source]
In this example, the Chain of Responsibility pattern is responsible for displaying contextual help information for active GUI elements. He keeps quoting lengthy excerpts from the manual, refusing to listen to your comments. After hearing the phrase “have you tried turning the computer off and on again? ” for the 10th time, you demand to be connected to a proper engineer.
If the Team Leader has authority to take action on that request, he will process the and give the result (approved/ denied). If he does not have the authority, he will pass it to the next person (Project Leader) in the chain. Please create a class file named Handler.cs and copy and paste the following code. Here, you can see that we created one variable of type Handler (i.e., NextHandler), and this variable will hold the reference of the Next Handler. If this is not clear now, don’t worry; you will understand the need for this variable after a while. The concrete handler classes will implement this abstract DispatchNote() method.
Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. Now we will define the ATM withdrawal process in order to define the use of paper currency dispenser. We will arrange the paper currency dispensers in higher to lower face value order. About the Author Marcin Zarębski is an experienced back-end developer, specializing in. He has amazing skills in building complex backend solutions and infrastructure. Marcin is also interested in space exploration and the role of computer science in it.
If a particular object cannot handle that request, it will pass the request to the next object in that chain. In simple words, we can say that the chain of responsibility design pattern creates a chain of receiver objects for a given request. In this design pattern, normally, each receiver contains a reference to the next receiver. If one receiver cannot handle the request, it passes the same request to the next receiver, and so on.
Comments
Post a Comment