Chain of Responsibility Design Pattern in Java
Table Of Content
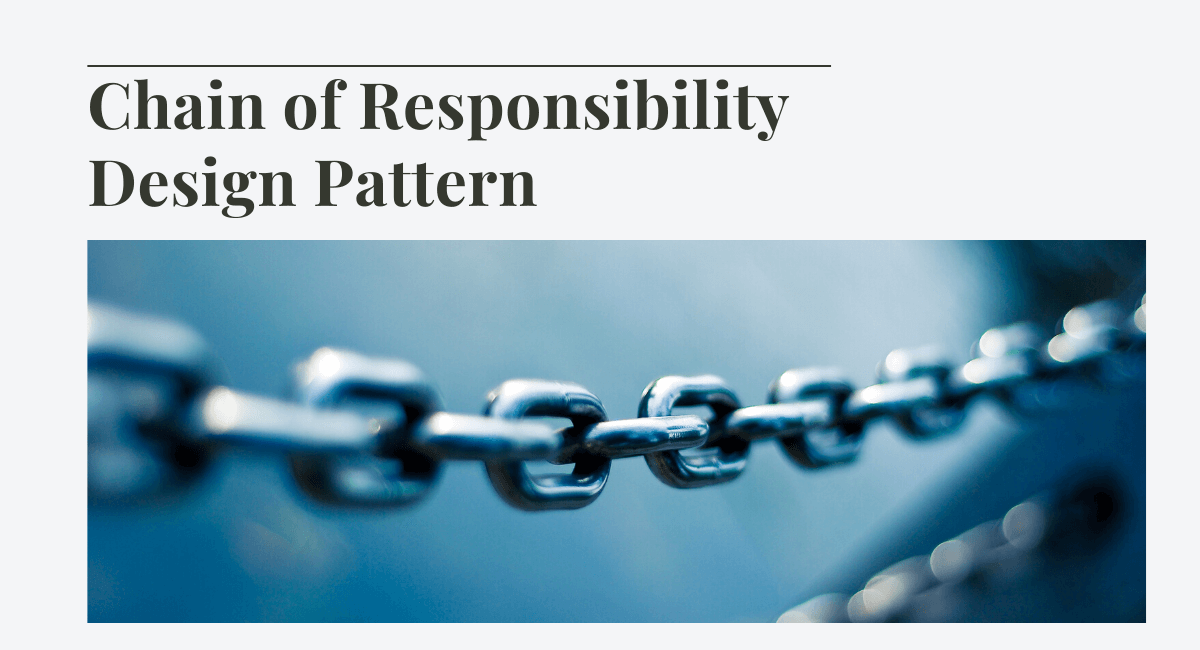
This makes the pattern suitable for scenarios where multiple, potentially different, requests need to be handled concurrently. The Chain of Responsibility pattern can potentially impact performance, as a request might need to be passed through several handlers before it is processed. However, this impact is usually negligible unless the chain is extremely long or the processing within each handler is complex. The pattern’s benefits in terms of code flexibility and maintainability often outweigh any minor performance concerns.
Step 1: Abstract Middleware Base
We have created an abstract class AbstractLogger with a level of logging. Then we have created three types of loggers extending the AbstractLogger. Each logger checks the level of message to its level and print accordingly otherwise does not print and pass the message to its next logger. For example, event handling mechanism in windows OS where events can be generated from either mouse, keyboard or some automatic generated events.
Structural Software Design Patterns in C++
The event is processed by the first element in the chain that’s capable of handling it. This example is also noteworthy because it shows that a chain can always be extracted from an object tree. The Chain of Responsibility is focused on the sequential processing of requests, while the Mediator pattern is concerned with centralizing and managing communication between objects. The Chain of Responsibility pattern is composed of key components and structured classes that work harmoniously to efficiently manage the flow of requests. The problem statement is to design a system for support service system consisting of front desk, supervisor, manager and director. If front desk is able to solve the issue, it will; otherwise will pass to supervisor.
How to Implement
Building and operating a secure online service - NCSC.GOV.UK - National Cyber Security Centre
Building and operating a secure online service - NCSC.GOV.UK.
Posted: Wed, 02 Mar 2022 08:00:00 GMT [source]
It’s crucial that all handler classes implement the same interface. Each concrete handler should only care about the following one having the execute method. This way you can compose chains at runtime, using various handlers without coupling your code to their concrete classes. In our example with ordering systems, a handler performs the processing and then decides whether to pass the request further down the chain.
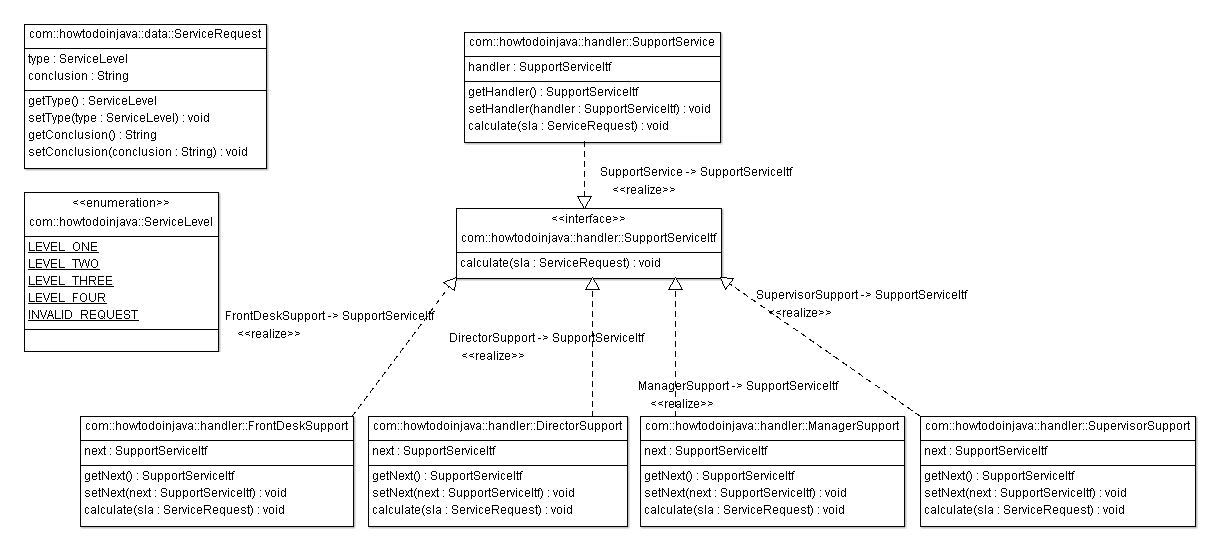
Contribute to nisal-kumara/krish-lp-training development by creating an account on GitHub.
Chain of responsibility pattern is used to achieve loose coupling in software design where a request from client is passed to a chain of objects to process them. Then the object in the chain will decide themselves who will be processing the request and whether the request is required to be sent to the next object in the chain or not. This way, we can easily add or remove handlers without modifying the client code, providing flexibility and scalability in handling customer requests. These handlers, like links in a chain, process the request or pass it to the next handler in line.
And the third example – it's the code for an ATM that checks if it can dispense cash using a finite number of notes inside the machine. In this case, we will have just one implementation of an abstract class, but we will define a chain consisting of three links using that one class. The Project Leader will check whether he can approve the leave or not. As the leave is for 25 days, the Project Leader will not approve the leave as his capacity is up to 20 days.
By separating sender and receiver objects, this pattern simplifies the process of modifying and expanding the system without affecting other parts of the code. It is especially useful in scenarios where numerous objects need to respond to requests in a hierarchical or sequential manner, such as in authentication, authorization, validation, or event processing. Thus, developers can seamlessly create flexible systems by leveraging the Chain of Responsibility pattern. The Chain of Responsibility pattern is a powerful design pattern that promotes scalability, flexibility, and maintainability in software systems. As you can see, the “applyLeave” method contains the core logic of these classes. So, whenever a leave request receives to the handler, it will pass to the Team Leader.
The request can be passed along a chain of objects until it is processed. This promotes loose coupling and flexibility in code structure, making it easier to add, remove, or reorder the objects in the chain without affecting the sender. The pattern allows multiple objects to handle the request without coupling sender class to the concrete classes of the receivers. The chain can be composed dynamically at runtime with any handler that follows a standard handler interface. The request will receive through a handler and the objects in the chain will decide themselves who will going to process that request. Each of these objects contain certain type of commands to handle that request.
The Team Leader will check whether he can approve the leave or not. As the leave is for 25 days and he can only approve up to 10 days, he will forward the request to the Project Leader. Please look at the following diagram to understand the Chain of Responsibility Design Pattern. On the right side, we have multiple receivers chained together (i.e., receiver 1 has a reference to receiver 2, receiver 2 has a reference to receiver 3, and so on).
Assuming the request contains the right data, all the handlers can execute their primary behavior, whether it’s authentication checks or caching. We create two concrete handlers, ConcreteHandlerA and ConcreteHandlerB, which implements the handle_request method to process or pass requests. In this step, we define an abstract base class Handler with a method handle_request. The Chain of Responsibility Design Pattern is a behavioral pattern that provides a solution for passing requests along a chain of handlers.
So, what the project leader will do is he will pass the request to HR, and HR will check and approve the leave. Here, we will create four handlers (TwoThousandHandler, FiveHundredHandler, TwoHundredHandler, and HundredHandler) to handle the respective currency. Create a class file named TwoThousandHandler.cs and copy and paste the following code.
We implement the RequestHandler class responsible for the final request processing and provides client code to create and configure the middleware chain. This pattern is recommended when multiple objects can handle a request and the handler doesn’t have to be a specific object. Please note that that a request not handled at all by any handler is a valid use case. When developing software, you may encounter situations where specific tasks need to be performed, but you’re uncertain about who will carry out these tasks. In such cases, the JavaScript Chain of Responsibility design pattern comes to the rescue.
Comments
Post a Comment